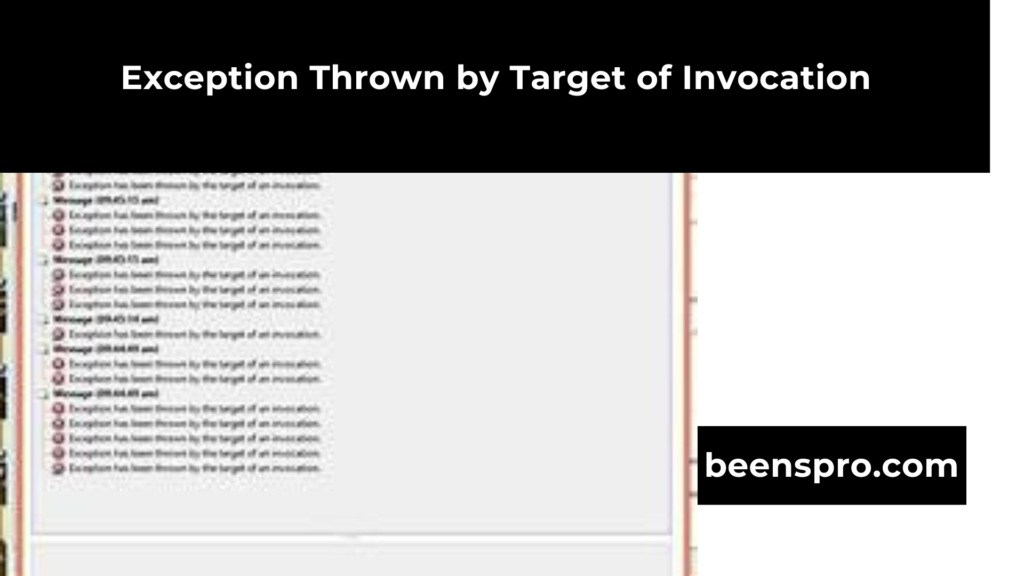
What is an Exception Has Been Thrown by the Target of an Invocation Error?
The “Exception Has Been Thrown by the Target of an Invocation” error is a common exception in programming, specifically in environments that utilize reflection or dynamic method invocations. This error occurs when a method invoked by reflection or another invocation mechanism throws an exception. Instead of directly reporting the error from the method itself, the exception is propagated as part of the invocation process, resulting in this error message.
In simpler terms, when a method throws an error during its execution, and that method was invoked dynamically (via reflection or another invocation technique), the thrown exception appears as an “Invocation Target Exception.” This issue usually arises in languages like Java, C#, and other object-oriented programming languages that make use of reflection or dynamic method calls.
Understanding the Invocation Target Exception Error
What is the Invocation Target Exception?
The “Invocation Target Exception” is a type of runtime exception that occurs during method invocation. It is a reflection exception triggered when a method call made using reflection throws an exception. Reflection is a technique used in Java and C# where methods, fields, or constructors are dynamically called or accessed at runtime. When a method called by reflection throws an exception, it is encapsulated in an “Invocation Target Exception” instead of being thrown directly.
Why Does an “Exception Thrown by Target of Invocation” Occur?
There are several causes of this exception, and understanding the root cause is crucial to fixing it. Some common reasons for the occurrence of this exception include:
- Method Invocation with Incorrect Parameters – If the method parameters passed through reflection do not match the method’s expected parameters, the invocation will fail and throw an exception.
- Errors in the Target Method – The method being invoked may throw an exception itself, such as a null pointer exception or an array index out-of-bounds exception, causing the invocation to fail.
- Invocation on a Non-Existing Method – This happens when a method does not exist in the class or interface being reflected upon. Calling an incorrect method name or using the wrong signature will lead to invocation errors.
Understanding the causes of invocation target exceptions is crucial for diagnosing and resolving them in your code.
How to Handle an Invocation Target Error?
Fixing Invocation Target Exception in Java: Step-by-Step Guide
Invocation target exceptions in Java often occur when using reflection. To resolve invocation target exceptions in Java, follow these steps:
- Review the Stack Trace: Java exceptions typically provide a stack trace that shows where the error occurred. In the case of invocation target exceptions, look at the method name and the class where the error originated. This will help you pinpoint the reflection call that failed.
- Check Method Parameters: Ensure that the parameters passed to the method match the method signature. If you are passing incorrect parameters, the method invocation will fail.
- Handle Exceptions in the Target Method: The target method might be throwing an exception that leads to the invocation failure. Wrap the target method inside a try-catch block to handle the exception and prevent it from propagating to the invocation layer.
- Debugging with Reflection: Use Java debugging tools such as IntelliJ IDEA or Eclipse to step through the code and observe the reflection call process. This will allow you to inspect parameter values, method signatures, and more, making it easier to resolve the error.
Fixing Invocation Target Exception in C#: A Practical Approach
In C#, fixing an invocation target exception follows a similar process but involves different debugging tools:
- Use Visual Studio Debugger: Visual Studio’s debugging tool is powerful for tracing method invocation errors. It helps inspect method parameters, the call stack, and local variables, making it easier to pinpoint the problem.
- Correct Parameter Types: Like Java, ensure the method parameters match the signature of the method you are invoking. In C#, reflection allows for dynamic method invocation, and incorrect parameters will cause errors.
- Catch Exceptions in the Target Method: When a target method throws an exception, catch it in the calling code to avoid triggering an invocation target exception. Wrap the method call in a try-catch block to handle potential errors.
- Log the Exception: Use logging to record the exception thrown by the target method. This will help in identifying the exact issue, especially when dealing with reflection-based invocations.
Best Practices for Exception Handling During Method Invocation
Best Practices for Managing “Exception Thrown” Errors in Programming
To avoid encountering “Exception Thrown” errors during method invocation, it is essential to adopt some best practices:
- Use Try-Catch Blocks Effectively: Enclose your method invocations inside try-catch blocks to handle any unexpected exceptions that might occur. Catching exceptions provides a controlled environment and prevents the application from crashing.
- Validate Method Parameters: Before invoking any method, ensure that the parameters are valid. Validate data types, object references, and any values passed to the method to avoid mismatches that lead to errors.
- Test Code Thoroughly: Thorough testing, including unit and integration tests, can help detect potential invocation issues. Always write test cases that simulate method invocations to catch errors early.
- Use Reflection Safely: Avoid overusing reflection, as it can make your code more prone to errors. Use reflection only when necessary, and always check if the method exists before calling it dynamically.
Reflection API: Handling Exceptions Effectively
The Reflection API is a powerful tool but can introduce complexities, especially when exceptions are thrown by the invoked methods. Here are some tips for handling exceptions effectively when using reflection:
- Use Access Control Checks: Before invoking a method using reflection, ensure the method is accessible. Use reflection methods like setAccessible(true) to ensure the method can be accessed if it is private.
- Handle Exceptions in Target Methods: Reflection allows invoking methods dynamically, but if the target method throws an exception, it will be wrapped inside an InvocationTargetException. Always catch and handle these exceptions to prevent crashes.
- Optimize Reflection Use: Use reflection sparingly. If reflection is causing frequent errors, refactor the code to avoid dynamic method invocation whenever possible.
Troubleshooting and Debugging Invocation Errors
How to Debug an Invocation Target Exception?
Debugging an Invocation Target Exception is a systematic process that helps identify the root cause. Here’s how you can troubleshoot this error:
- Use a Debugger: Step through the code using a debugger to see the call stack and method arguments at runtime. This will show you where the invocation fails and what arguments were passed to the method.
- Check for Null References: Ensure that the object you are invoking the method on is not null. Null references are a common cause of invocation errors, particularly when using reflection.
- Log the Exception: Always log the exception thrown by the target method. This will give you a more detailed insight into why the invocation failed, helping you fix the underlying issue.
- Examine Method Signatures: Verify that the method signature you’re using matches the target method’s expected parameters. A mismatch will result in an invocation target exception.
Utilizing Debugging Tools for Efficient Invocation Error Handling
Several debugging tools can be employed to efficiently handle invocation target errors:
- IntelliJ IDEA: Use IntelliJ IDEA’s debugger to examine stack traces, method parameters, and invocation points in real time.
- Visual Studio: Visual Studio offers advanced debugging features for both managed and unmanaged code, allowing you to track invocation errors effectively.
- Eclipse: In Java, Eclipse provides powerful tools for debugging reflection-based code, offering breakpoints and step-through functionality.
Preventing Invocation Target Exceptions in Future Code
How to Prevent Invocation Target Failures in Your Code?
To ensure you don’t run into invocation target errors in the future, consider these preventive measures:
- Use Type-Safe Methods: Whenever possible, use type-safe methods and avoid reflection. If reflection is necessary, ensure type checks are in place to validate arguments and method signatures.
- Validate Inputs: Always validate the parameters passed to methods, particularly when working with reflection. This will reduce the chances of parameter mismatch causing invocation failures.
- Write Robust Tests: Ensure that all methods invoked via reflection or dynamic methods are tested thoroughly. This can help catch errors before they happen in production environments.
Best Programming Practices for Avoiding Reflection Errors
Reflection can introduce complexity and errors into your code. To avoid invocation target exceptions when using reflection:
- Check Method Existence: Before calling a method using reflection, ensure the method exists in the target class with the correct parameters.
- Use Reflection Judiciously: Avoid overusing reflection. Whenever possible, rely on regular method invocations instead of dynamic calls via reflection.
Conclusion
In conclusion, understanding and handling the Invocation Target Exception is essential for developers working with dynamic method invocations or reflection. By following best practices, using proper debugging techniques, and validating method signatures and parameters, you can minimize the chances of encountering this exception and improve the reliability of your code.
FAQs
What does it mean when an “Exception Has Been Thrown by the Target of an Invocation” error occurs?
This error occurs when a method called dynamically using reflection throws an exception. The exception is propagated as part of the invocation process, appearing as an Invocation Target Exception.
How can I debug an “Exception Thrown by the Target of an Invocation” in C#?
Use Visual Studio’s debugger to inspect the call stack and parameters. This helps you find the point where the invocation fails and identify which method or argument causes the issue.
How can I prevent invocation target exceptions in Java?
Validate the method parameters before invoking them, use try-catch blocks, and test the methods thoroughly to ensure they behave correctly under reflection-based invocation.
What are the common causes of an invocation target exception in Java?
The most common causes include incorrect method parameters, non-existent methods, or exceptions thrown inside the target method.
What tools can help debug invocation target exceptions?
Tools like IntelliJ IDEA, Eclipse, and Visual Studio provide debugging features that allow you to inspect the call stack and method parameters, helping to pinpoint invocation target exceptions.